Java Interview questions - Updated March 2022
Iterate hashmap
Map<String, Object> map = ...;
for (String key : map.keySet()) {
// ...
}
for (Object value : map.values()) {
// ...
}
for (Map.Entry<String, Object> entry : map.entrySet()) {
String key = entry.getKey();
Object value = entry.getValue();
// ...
}
HashMap itself doesn't maintain insertion order - but LinkedHashMap does, so use that instead.
map() vs flatmap()
Stream.flatMap, as it can be guessed by its name, is the combination of a map and a flat operation. That means that you first apply a function to your elements, and then flatten it. Stream.map only applies a function to the stream without flattening the stream.
To understand what flattening a stream consists in, consider a structure like [ [1,2,3],[4,5,6],[7,8,9] ] which has "two levels". Flattening this means transforming it in a "one level" structure : [ 1,2,3,4,5,6,7,8,9 ].
@ExceptionHandler
@ExceptionHandler works at the Controller level and it is only active for that particular Controller, not globally for the entire application.
HandlerExceptionResolver
This will resolve any exception thrown by the application. It is used to resolve standard Spring exceptions to their corresponding HTTP Status Codes. It does not have control over the body of the response, means it does not set anything to the body of the Response.It does map the status code on the response but the body is null.
@ControllerAdvice
@ControllerAdvice used for global error handling in the Spring MVC application.It also has full control over the body of the response and the status code.
How to notice a memory leak in Java?
An OutOfMemoryError (OOM) is a sign of a memory leak. Further, if the application’s memory utilization is on the rise the data processing remains the same, one may be dealing with a memory leak. Similarly, performance problems with large data sets is indicative of a memory leak. Tools like Java VisualVM help one analyze code and identify memory leaks.
how does abstraction differ from encapsulation?
While abstraction focuses on an object’s behavior, encapsulation focusses on the implementation of an object’s behavior. Abstraction hides unwanted information whereas encapsulation hides the data into a single unit, protecting the information from outside influence.
print the content of a multidimensional array
Arrays.deepToString(obj)
Java Reflection enables us to inspect classes, interfaces, fields, and methods at runtime. We can do it without knowing the names of the classes, methods, at compile time.
For example, all objects in Java have the method getClass(), which lets you determine the object's class even if you don't know it at compile time
Fail-Fast :
- Iterators immediately throw ConcurrentModificationException if structural modification(add, update, delete) happens.
- Example : ArrayList, HashMap, TreeSet
Fail-Safe :
- Here Iterators not throw any exception because they operate on the clone of the collection, not original one. So, that they are fail-safe iterators.
- Example : CopyOnWriteArrayList, ConcurrentHashMap
JavaBeans:
At a basic level, JavaBeans are simply Java classes which adhere to certain coding conventions. Specifically, classes that
- have public default (no argument) constructors
- allow access to their properties using accessor (getter and setter) methods
- implement java.io.Serializable
Spring Beans:
A Spring bean is basically an object managed by Spring. More specifically, it is an object that is instantiated, configured and otherwise managed by a Spring Framework container. Spring beans are defined in Spring configuration files (or, more recently, with annotations), instantiated by Spring containers, and then injected into applications.
Note that Spring beans need not always be JavaBeans. but every java bean can be a spring bean
cookie is a small piece of data that the Web server sends to the browser. The browser stores the cookies for each Web server in a local file. Cookies provide a reliable mechanism for websites to remember stateful information or to record the browsing activity of users.
The differences between the session and a cookie are:
- The session should work irrespective of the settings on the client’s browser. The client can choose to disable cookies. However, the sessions still work because the client has no ability to disable them on the server-side.
- The session and cookies are also different in the amount of information they can store. The HTTP session can store any Java object, while a cookie can only store String objects.
- Double brace initialization creates an anonymous class derived from the specified class (the outerbraces), and provides an initialiser block within that class (the innerbraces). e.g.
new ArrayList<Integer>() {{
add(1);
add(2);
}};
Marshalling: When a client invokes a method that accepts parameters on a remote object, it bundles the parameters into a message before sending it over the network. These parameters can be of primitive type or objects. When the parameters are of primitive type, they are put together and a header is attached to it. If the parameters are objects, then they are serialized. This process is called marshalling.
Unmarshalling: The packed parameters are unbundled at the server-side, and then the required method is invoked. This process is called unmarshalling.
marshalling: converting java objects to xml
unmarshalling: converting xml to java objects
CountDownLatch maintains a count of tasks whereas CyclicBarrier maintains a count of threads.
What are the best coding practices that you learned in Java?
Answer. If you are learning and working on a programming language for a couple of years, you must surely know a lot of its best practices. The interviewer just checks by asking a couple of them, that you know your trade well. Some of the best coding practices in Java can be:
- Always try to give a name to the thread, this will immensely help in debugging.
- Prefer to use the StringBuilder class for concatenating strings.
- Always specify the size of the Collection. This will save a lot of time spent on resizing the size of the Collection.
- Always declare the variables as private and final unless you have a good reason.
- Always code on interfaces instead of implementation.
- Always provide dependency on the method instead they get it by themselves. This will make the coding unit testable.
What is the difference between creating an object using new operator and Class.forName().newInstance()?
The new operator statically creates an instance of an object. Whereas, the newInstance() method creates an object dynamically. While both the methods of creating objects effectively do the same thing, we should use the new operator instead of Class.forName(‘class’).getInstance().
The getInstance() method uses the Reflection API of Java to lookup the class at runtime. But, when we use the new operator, Java Virtual Machine will know beforehand that we need to use that class and therefore it is more efficient.
Why is the Char array preferred over String for storing passwords?
As we know that String is immutable in Java and stored in the String pool. Once we create a String, it stays in the String pool until it is garbage collected. So, even though we are done with the password it is still available in memory for a longer duration. Therefore, there is no way to avoid it.
It is clearly a security risk because anyone having access to a memory dump can find the password as clear text. Therefore, it is preferred to store the password using the char array rather than String in Java.
Both System.out and System.err represent the Monitor by default. Hence they are used to send or write data or results to the monitor. System.out displays normal messages and results on the monitor whereas System.err displays the error messages. System.in represents an InputStream object, which by default represents a standard input device, that is, the keyboard.
What is a metaspace?
The Permanent Generation or PermGen space has been completely removed and replaced by a new space called Metaspace. The result of removing the PermGen removal is that the PermSize and MaxPermSize JVM arguments are ignored and we will never get a java.lang.OutOfMemoryError: PermGen error.
Does the garbage collector of Java guarantee that a program will not run out of memory?
There is no guarantee that using a Garbage collector will ensure that the program will not run out of memory. As garbage collection is an automatic process, programmers need not initiate the garbage collection process explicitly in the program. A Garbage collector can also choose to reject the request and therefore, there is no guarantee that these calls will surely do the garbage collection. Generally, JVM takes this decision based on the availability of space in heap memory.
- Stack Segment: The stack segment contains the local variables and reference variables. Reference variables hold the address of an object in the heap segment.
- Heap Segment: The heap segment contains all the objects that are created during runtime. It stores objects and their attributes (instance variables).
- Code Segment: The code segment stores the actual compiled Java bytecodes when loaded.
Does not overriding hashCode() method have any impact on performance?
A poor hashCode() function will result in the frequent collision in HashMap. This will eventually increase the time for adding an object into HashMap. But, from Java 8 onwards, the collision will not impact performance as much as it does in earlier versions. This is because after crossing a threshold value, the linked list gets replaced by a binary tree, which will give us O(logN) performance in the worst case as compared to O(n) of a linked list.
Dynamic Method Dispatch is also called runtime polymorphism. It is a method in which the overridden method is resolved during the runtime, not during the compilation of the program. More specifically, the concerned method is called through a reference variable of a superclass.
difference between sleep() and wait()
sleep() does not release the lock while wait method release the lock. sleep() method is present in java.lang.Thread class while wait() method is present in java.lang.Object class.
What will happen if we put a key object in a HashMap which is already there?
If you put the same key again then it will replace the old mapping because HashMap doesn't allow duplicate keys.
Factory design pattern is the most commonly used pattern in Java. They belong to Creational Patterns because it provides means of creating an object of different instances. Here, the logic of object creation is not exposed to the client. It is implemented by using a standard interface.
Is it necessary to declare all immutable objects as final?
This is not necessary. The functionality of achieving immutability can also be achieved by defining the members of a class as private and not providing any setter methods to modify/update the values. Any references to the members should not be leaked and the only source of initializing the members should be by using the parameterized constructor.
We should note that the variables declared as final only takes care of not re-assigning the variable to a different value. The individual properties of an object can still be changed.
IntegerCache (-128 to 127 are stored as cache)
Integer.java class in Java has a private inner class called IntegerCache.java which helps to cache the Integer objects ranging from -128 to 127. All the numbers lying between this range are cached internally by the Integer class.
Creating string using the new operator ensures that the String is created in the heap alone and not into the string pool. Whereas, creating string using literal ensures that the string is created in the string pool. String pool exists as part of the perm area in the heap. This ensures that the multiple Strings created using literal having same values are pointed to one object and prevents duplicate objects with the same value from being created.
The Heap Space contains all objects are created, but Stack contains any reference to those objects.
Is it possible to override a method to throw RuntimeException from throwing NullPointerException in the parent class?
Yes, this is possible. But it is not possible if the parent class has a checked Exception. This is due to the below rule of method overriding in cases of checked exceptions:
The method that wants to override a parent class method can not throw a higher Exception than the overridden method.
This means that if the overridden method is throwing IOException, then the overriding child class method can only throw IOException or its sub-classes. This overriding method can not throw a higher Exception than the original or overridden method
System.out.println(0.1*3 == 0.3); -> Prints false
System.out.println(0.1*2 == 0.2); -> Prints true
This expectation mismatch is due to the error that occurs while rounding float-point numbers and the fact that in Java, only the floating-point numbers that are powers of 2 are represented accurately by the binary representation. The rest of the numbers should be rounded to accommodate the limited bits as required.
How would you help a colleague with lesser Java experience who has trouble in serializing a class?
I would first check if the colleague has implemented the java.io.serializable interface. If this is done, I will check if they are trying to serialize only non-static members since the static members cannot be serialized. I would also check if the fields that are not serialized are defined as transient accidentally
What is the result of the below code and Why?
public class TestClass {
public static void main(String[] args) {
someMethod(null);
}
public static void someMethod(Object o) {
System.out.println("Object method Invoked");
}
public static void someMethod(String s) {
System.out.println("String method Invoked");
}
}
The output of this code is “String method Invoked”. We know that null is a value that can be assigned to any kind of object reference type in Java. It is not an object in Java. Secondly, the Java compiler chooses the method with the most specific parameters in method overloading. this means that since the String class is more specific, the method with String input parameter is called.
How would you help a colleague with lesser Java experience who has trouble in serializing a class?
I would first check if the colleague has implemented the java.io.serializable interface. If this is done, I will check if they are trying to serialize only non-static members since the static members cannot be serialized. I would also check if the fields that are not serialized are defined as transient accidentally
How is the classpath variable different from the path variables?
The classpath variables are specific to the Java executables and are used for locating the class files. Whereas, the path variable is a variable present in the operating system and is used for locating the system executables
hashCode() and equals() contract
If these methods are not implemented properly, then there are chances where two keys would produce the same output for these methods resulting in inaccuracy and a wrong key’s value might get updated at the time of updation. Hence, it is very much important to implement the hashCode and the equals method correctly. This can be done properly if we follow the hashCode-equals contract. The contract states that
If two objects are equal, then the hashCode method should produce the same result for both objects.
To ensure this, we have to override the hashCode() method whenever we override the equals() method
What do you understand by the ... in the below method parameters?
public void someMethod(String... info){
// method body
}
The 3 dots feature was started in Java 5 and the feature is known as varargs (variable arguments). This simply means that the method can receive one or more/multiple String arguments
How is Collection different from Collections in Java?
Collection is an interface whereas Collections is a java utility class containing only static methods that can operate on collections like ArrayList, Set, Queue, etc., and both belong to Collection Framework and are present in the java.util package.
Volatile Variable | Transient Variable |
---|---|
The volatile keyword against the variable indicates that the content of the variable is stored in the main memory and every read of the variable should be done from the main memory and not the CPU cache and every write should be written to the main memory and not just to the CPU cache. | Transient is used when we do not want the variable to be serialized. |
Volatile ensures that the JVM does not re-order the variables and ensures that the synchronization issues are avoided. | Transient provides flexibility and control over the attributes of objects from being serialized. |
Volatile variables do not have any default values. | Transient variables are initialized with default value corresponding to the data type at the time of deserialization. |
Volatile variables can be used along with the static keyword. | Transient variables cant be used along with static keywords because the static variables are class-level variables and not related to the individual instances. This matters during serialization. |
Volatile variables can be used along with the final keyword. | It is not recommended to use final with transient variables as it would cause problems for re-initializing the variables once the values are populated by default at the time of deserialization. |
Why String is immutable?
- Security: parameters are typically represented as String in network connections, database connection urls, usernames/passwords etc. If it were mutable, these parameters could be easily changed.
- Synchronization and concurrency: making String immutable automatically makes them thread safe thereby solving the synchronization issues.
- Caching: when compiler optimizes your String objects, it sees that if two objects have same value (a="test", and b="test") and thus you need only one string object (for both a and b, these two will point to the same object).
- Class loading: String is used as arguments for class loading. If mutable, it could result in wrong class being loaded (because mutable objects change their state).
That being said, immutability of String only means you cannot change it using its public API. You can in fact bypass the normal API using reflection. See the answer here.
In your example, if String was mutable, then consider the following example:
String a="stack";
System.out.println(a);//prints stack
a.setValue("overflow");
System.out.println(a);//if mutable it would print overflow
Abstraction | Encapsulation |
---|---|
Abstraction is the process or method of gaining information. | While encapsulation is the process or method to contain the information. |
In abstraction, problems are solved at the design or interface level. | While in encapsulation, problems are solved at the implementation level. |
Abstraction is the method of hiding unwanted information. | Whereas encapsulation is a method to hide the data in a single entity or unit along with a method to protect information from outside. |
We can implement abstraction using abstract classes and interfaces. | Whereas encapsulation can be implemented using by access modifier i.e. private, protected, and public. |
In abstraction, implementation complexities are hidden using abstract classes and interfaces. | While in encapsulation, the data is hidden using methods of getters and setters. |
The objects that help to perform abstraction are encapsulated. | Whereas the objects that result in encapsulation need not be abstracted. |
equals() | == |
---|---|
This is a method defined in the Object class. | It is a binary operator in Java. |
This method is used for checking the equality of contents between two objects as per the specified business logic. | This operator is used for comparing addresses (or references), i.e checks if both the objects are pointing to the same memory location. |
- JIT stands for Just-In-Time and it is used for improving the performance during run time. It does the task of compiling parts of byte code having similar functionality at the same time thereby reducing the amount of compilation time for the code to run.
feature of the new Date and Time API in Java 8?
- Immutable classes and Thread-safe
- Timezone support
- Fluent methods for object creation and arithmetic
- Addresses I18N issue for earlier APIs
- Influenced by popular joda-time package
A stream is an abstraction to express data processing queries in a declarative way.
ClassNotFoundException is an exception that occurs when you try to load a class at run time using Class.forName() or loadClass() methods and mentioned classes are not found in the classpath.
NoClassDefFoundError is an error that occurs when a particular class is present at compile-time but was missing at run time.
what is Method Reference?
Method reference is a compact way of referring to a method of functional interface. It is used to refer to a method without invoking it. :: (double colon) is used for describing the method reference. The syntax is class::methodName
How does a lambda expression relate to a functional interface?
Lambda expression is a type of function without a name. It may or may not have results and parameters. It is known as an anonymous function as it does not have type information by itself. It is executed on-demand. It is beneficial in iterating, filtering, and extracting data from a collection.
pre-defined functional interfaces from previous Java versions are Runnable, Callable, Comparator, and Comparable. While Java 8 introduces functional interfaces like Supplier, Consumer, Predicate
In terms of web apps, very crudely speaking, authentication is when you check login credentials to see if you recognize a user as logged in, and authorization is when you look up in your access control whether you allow the user to view, edit, delete or create content.
Authentication is the process of ascertaining that somebody really is whom they claim to be.
Authorization refers to rules that determine who is allowed to do what. E.g. Adam may be authorized to create and delete databases, while Usama is only authorized to read.
newly added features in Java 8?
Feature Name | Description |
---|---|
Lambda expression | A function that can be shared or referred to as an object. |
Functional Interfaces | Single abstract method interface. |
Method References | Uses function as a parameter to invoke a method. |
Default method | It provides an implementation of methods within interfaces enabling 'Interface evolution' facilities. |
Stream API | Abstract layer that provides pipeline processing of the data. |
Date Time API | New improved joda-time inspired APIs to overcome the drawbacks in previous versions |
Optional | Wrapper class to check the null values and helps in further processing based on the value. |
Nashorn, JavaScript Engine | An improvised version of JavaScript Engine that enables JavaScript executions in Java, to replace Rhino. |
The version which declares throws Exception will require the calling code to handle the exception, while the version which explicitly handles it will not.
valueOf(String) returns a new Integer() object whereas parseInt(String) returns a primitive int.
Once return is executed, the rest of the code won't be executed.
A functional interface cannot extend another interface with abstract methods as it will void the rule of one abstract method per functional interface.
Lazy Evaluation in Streams
In Java 8 streams the intermediate operations are not evaluated until a terminal operation is invoked.
In the case of a serial stream, the main thread is doing all the work. Meanwhile, in the case of a parallel stream, two threads are spawned simultaneously, and the stream internally uses ForkJoinPool to create and manage threads. Parallel streams create a ForkJoinPool instance via the static ForkJoinPool.commonPool() method.
The parallel stream makes use of all available CPU cores and processes the tasks in parallel. If the number of tasks exceeds the number of cores, then the remaining tasks wait for the currently running tasks to complete.
use parallel stream only when:
- We have a large amount of data to process.
- We already have a performance problem in the first place.
- All the shared resources between threads need to be synchronized properly otherwise it might produce unexpected results.
- Use the NQ formula to decide if you want to use parallelism.
NQ Model:
N x Q > 10000
where,
N = number of data items
Q = amount of work per item
Collectors.summingInt
This method returns a Collector that produces the sum of an integer-valued function applied to the input elements.
counting()
This function returns a Collector that counts the number of the input elements.
Matching Operations in Stream.
1) anyMatch()
2) allMatch()
3) noneMatch()
Slicing Operations in Stream
The slicing operations are intermediate operations, and, as the name implies, they are used to slice a stream.
- distinct()
- limit()
- skip()
According to Oracle, “Java 8 Optional works as a container type for the value which is probably absent or null. Java Optional is a final class present in the java.util package.”
The benefit of Optional<T> is not that we are saved from applying a null check. The benefit is that Optional<T> class provides us lots of utility methods that we can apply to our wrapped objects.
If while creating the Optional, you are not sure if the value is null or not null, then use the ofNullable() method.
Method references are shortened versions of lambda expressions that call a specific method.
The syntax to use static methods as method reference is ClassName::MethodName.
The syntax to use the instance method as a method reference is ReferenceVariable::MethodName
constructor reference is ClassName::new.
Reflection
Java Reflection is an API used to examine or modify the behavior of methods, classes, and interfaces at runtime. Using the Reflection API, we can create multiple objects in the Singleton class.
Prevent Singleton Pattern From Reflection
There are many ways to prevent Singleton pattern from Reflection API, but one of the best solutions is to throw a run-time exception in the constructor if the instance already exists. In this, we can not able to create a second instance.
Cloning
Using the "clone" method, we can create a copy of the original object; it's the same thing if we applied clone in the singleton pattern. It will create two instances: one original and another one cloned object. In this case, we will break the Singleton principle.
Prevent Singleton Pattern From Cloning
In the above code, it breaks the Singleton principle, i. e created two instances. To overcome the above issue, we need to implement/override the clone()
method and throw an exception CloneNotSupportedException
from the clone
method. If anyone tries to create a clone
object of Singleton
, it will throw an exception, as shown in the below code.
covariant Return types simply means returning own Class reference or its child class reference.
class Parent {
//it contain data member and data method
}
class Child extends Parent {
//it contain data member and data method
//covariant return
public Parent methodName() {
return new Parent();
or
return Child();
}
}
Synchronized HashMap:
- Each method is synchronized using an object level lock. So the get and put methods on synchMap acquire a lock.
- Locking the entire collection is a performance overhead. While one thread holds on to the lock, no other thread can use the collection.
ConcurrentHashMap was introduced in JDK 5.
- There is no locking at the object level,The locking is at a much finer granularity. For a ConcurrentHashMap, the locks may be at a hashmap bucket level.
- The effect of lower level locking is that you can have concurrent readers and writers which is not possible for synchronized collections. This leads to much more scalability.
- ConcurrentHashMap does not throw a ConcurrentModificationException if one thread tries to modify it while another is iterating over it.
- select max occurance of an element
SELECT itemCode , count(*) as MaxCount
FROM OrderProcessing
GROUP BY itemCode
HAVING count(*) =
-- count of most wanted item
(select top 1 count(*)
from OrderProcessing
group by itemCode
order by count(*) desc)
Sequential streams use a single thread to process the pipeline
Parallel streams enable us to execute code in parallel on separate cores
Parallel streams make use of the fork-join framework and its common pool of worker threads.
Callable is similar to Runnable but it returns a result and may throw an exception. Use them when you expect your asynchronous tasks to return result.
In order to create an immutable class, you should follow the below steps:
- Make your class final, so that no other classes can extend it.
- Make all your fields final, so that they’re initialized only once inside the constructor and never modified afterward.
- Don’t expose setter methods.
- When exposing methods which modify the state of the class, you must always return a new instance of the class.
- If the class holds a mutable object:
- Inside the constructor, make sure to use a clone copy of the passed argument and never set your mutable field to the real instance passed through constructor, this is to prevent the clients who pass the object from modifying it afterwards.
- Make sure to always return a clone copy of the field and never return the real object instance.
The API guarantees a stablesorting which Quicksortdoesn’t offer. However, when sorting primitive valuesby their natural order you won’t notice a difference as primitive values have no identity. Therefore, Quicksortcan used for primitive arrays and will be used when it is considered more efficient¹.
For objects you may notice, when objects with different identity which are deemed equal according to their equalsimplementation or the provided Comparatorchange their order. Therefore, Quicksortis not an option. So a variant of MergeSortis used, the current Java versions use TimSort. This applies to both, Arrays.sortand Collections.sort, though with Java 8, the Listitself may override the sort algorithms.
Differences that can help you understand the advantages of persist and save methods:
- First difference between save and persist is their return type. The return type of persist method is void while return type of savemethod is Serializable object.
- The persist() method doesn’t guarantee that the identifier value will be assigned to the persistent state immediately, the assignment might happen at flush time.
- The persist() method will not execute an insert query if it is called outside of transaction boundaries. While, the save() method returns an identifier so that an insert query is executed immediately to get the identifier, no matter if it are inside or outside of a transaction.
- The persist method is called outside of transaction boundaries, it is useful in long-running conversations with an extended Session context. On the other hand save method is not good in a long-running conversation with an extended Session context.
- persist is supported by JPA, while save is only supported by Hibernate.
If a JUnit test method is declared as "private", it compiles successfully. But the execution will fail. This is because JUnit requires that all test methods must be declared as "public".
SAX vs DOM
DOM stands for Document Object Model. The DOM parser does not rely on events. Moreover, it loads the whole XML document into memory to parse it. SAX is more memory-efficient than DOM.
DOM has its benefits, too. For example, DOM supports XPath. It makes it also easy to operate on the whole document tree at once since the document is loaded into memory.
SAX vs StAX
StAX is more recent than SAX and DOM. It stands for Streaming API for XML.
The main difference with SAX is that StAX uses a pull mechanism instead of SAX's push mechanism (using callbacks). This means the control is given to the client to decide when the events need to be pulled. Therefore, there is no obligation to pull the whole document if only a part of it is needed.
It provides an easy API to work with XML with a memory-efficient way of parsing.
Unlike SAX, it doesn't provide schema validation as one of its features.
Marshalling is the process of writing Java objects to XML file. Unmarshalling is the process of converting XML content to Java objects
String Interning is a method of storing only one copy of each distinct String Value, which must be immutable.
By applying String.intern() on a couple of strings will ensure that all strings having the same contents share the same memory
An Armstrong number is a positive n-digit number that is equal to the sum of the nth powers of their digits.
Questions: Differentiate between this() and super()
Answer:
this() | super() |
Represents the current instance of the class. | Represents the current instance of the parent/base class. |
It is used to call the default constructor of the same class | It is used to call the default constructor of the parent/base class. |
Accesses method of the current class | Accesses method of the base class |
Points current class instance | Points to the superclass instance. |
Must be the first line of the block | It must be the first line of the block. |
Question: Explain the concept of boxing, unboxing, autoboxing, and auto unboxing.
The process of creating an Integer from an int is called boxing. The reverse is called unboxing. Because having to box primitives every time you want to use them as Object is inconvenient, there are cases where the language does this automatically - that's called autoboxing.
Serialization is the process by which Java objects are converted into the byte stream.
The volatile keyword does not cache the value of the variable and always read the variable from the main memory.
- static volatile int var =5;
- It can be used as an alternative way of achieving synchronization in Java.
- All reader threads will see the updated value of the volatile variable after completing the write operation.
The transient keyword in Java is used to avoid serialization.
- DDL – Data Definition Language
- DML – Data Manipulation Language
- TCL – Transaction Control Language.
- DQL – Data Query Language
- DCL – Data Control Language
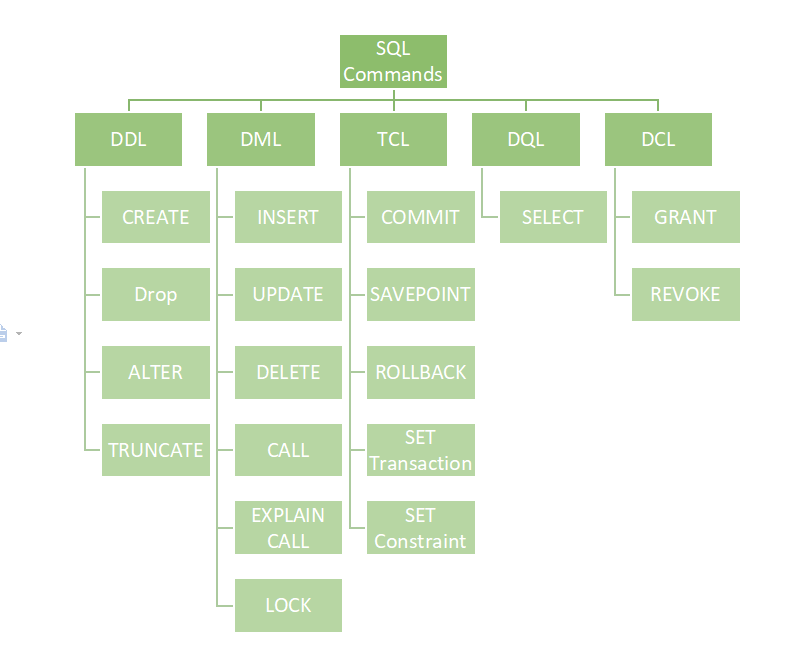
- Checked Exceptions – Classes that extend Throwable class, except Runtime exception and Error, are called checked exceptions. Such exceptions are checked by the compiler during the compile time. These types of exceptions must either have appropriate try/catch blocks or be declared using the throws keyword. ClassNotFoundException is a checked exception.
- Unchecked Exceptions – Such exceptions aren't checked by the compiler during the compile time. As such, the compiler doesn't necessitate handling unchecked exceptions. Arithmetic Exception and ArrayIndexOutOfBounds Exception are unchecked exceptions.
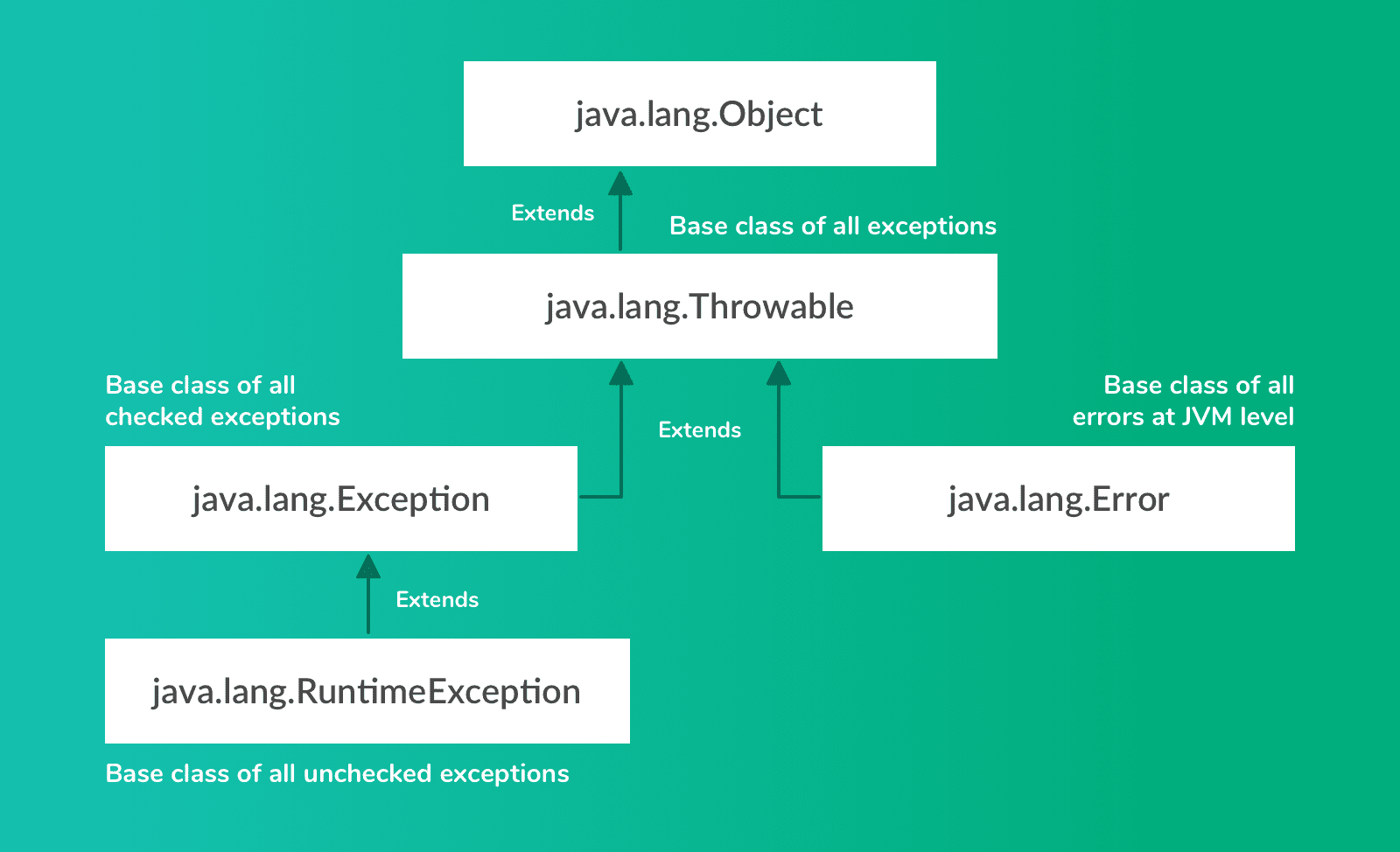
runtime exception can be caught, like any other exception.
The throws keyword is followed by a class, whereas the throw keyword is followed by an instance
Why is char[] preferred over String for passwords?
Strings are immutable. That means once you've created the String if another process can dump memory, there's no way (aside from reflection) you can get rid of the data before garbage collection kicks in.
With an array, you can explicitly wipe the data after you're done with it. You can overwrite the array with anything you like, and the password won't be present anywhere in the system, even before garbage collection.
== tests for reference equality (whether they are the same object).
.equals() tests for value equality (whether they are logically "equal").
Objects.equals() checks for null before calling .equals()
HashMap works on the principle of hashing
put(key, value): HashMap stores both key and value object as Map.Entry. Hashmap applies hashcode(key) to get the bucket. if there is collision ,HashMap uses LinkedList to store object.
get(key): HashMap uses Key Object's hashcode to find out bucket location and then call keys.equals() method to identify correct node in LinkedList and return associated value object for that key in Java HashMap.
When we try to insert any key in HashMap first it checks whether any other object present with same hashcode and if yes then it checks for the equals() method. If two objects are same then HashMap will not add that key instead it will replace the old value by new one.
Avoid raw types.
Raw types refer to using a generic type without specifying a type parameter.
For example:
A list is a raw type, while List<String> is a parameterized type.
A disadvantage of using the thread class is that it becomes impossible to extend any other classes.
Nonetheless, it is possible to overload the run() method in the class
An abstract class in Java is a class that can't be instantiated
if there is a collision, HashMap uses LinkedList to store objects
https://javarevisited.blogspot.com/2011/02/how-hashmap-works-in-java.html.
Question: How does Java enable high performance?
Answer: In the Just-in-Time compilation, the required code is executed at run time. Typically, it involves translating bytecode into machine code and then executing it directly. For enabling high performance, Java can make use of the Just-In-Time compilation. The JIT compiler is enabled by default in Java and gets activated as soon as a method is called. It then compiles the bytecode of the Java method into native machine code. After that, the JVM calls the compiled code directly instead of interpreting it. This grants a performance boost.
Question: Explain different types of typecasting?
Answer:
Different types of typecasting are:
- Implicit: Storing values from a smaller data type to a larger data type. It is automatically done by the compiler.
- Explicit: Storing the value of a larger data type into a smaller data type. This results in information loss:
Superclass is the existing class from which the new classes are derived while Subclass is the new class that inherits the properties and methods of the Superclass.
Questions: Differentiate between break and continue
Answer:
Break | Continue |
Used with both loop and switch statement | Used with only loop statements. |
It terminates the loop or switch block. | It does not terminate but skips to the next iteration. |
Static methods can not be overridden.
Constructors can be overloaded.
Question: Please explain Local variables and Instance variables in Java.
Answer: Variables that are only accessible to the method or code block in which they are declared are known as local variables. Instance variables, on the other hand, are accessible to all methods in a class. While local variables are declared inside a method or a code block, instance variables are declared inside a class but outside a method.
The problem with Hashtable is that synchronizing each method call (which is a not-insignificant operation) is usually the wrong thing. Either you don't need synchronization at all or, from the point of view of the application logic, you need to synchronize over transactions that span multiple method calls. Since it was impossible to simply remove the method-level synchronization from Hashtable without breaking existing code, the Collections framework authors needed to come up with a new class; hence HashMap. It's also a better name since it becomes clear that it's a kind of Map.
Builder pattern solves multiple constructors problem (telescopic constructor)
inner class (static)
- private instance variable
- default constructor
- private build method which returns new instance of outer class
- setters which returns instance of Builder Object (inner class object)
outer class:
- private instance variables
- private constructor with Builder class as parameter
- getters and toString using StringBuffer
Type casting is a way of converting data from one data type to another data type. This process of data conversion is also known as type conversion or type coercion. In Java, we can cast both reference and primitive data types. By using casting, data can not be changed but only the data type is changed
Highest Salary
SELECT * FROM employee
WHERE salary= (SELECT DISTINCT(salary)
FROM employee ORDER BY salary DESC LIMIT n-1,1);
We can declare a method as final, once you declare a method final it cannot be overridden.
Memory management Unlike some of its competitors (C and C++), Java provides automatic
memory management.
Why do we need Method Injection?
Method Injection should be used is when a Singleton bean has a dependency on the Prototype bean.
We know that the Spring beans can be created with a singleton or prototype scope.
Singleton: Instantiate only one object
Prototype: Instantiate a new object every time.
Spring container resolves the dependencies at instantiation time which means if any singleton bean has a dependency of any prototype bean, then a new object of prototype bean will be instantiated and injected into singleton bean at the time of instantiation of Singleton bean.
With this, the same prototype bean will always be supplied from singleton bean.
Confused? Consider a Singleton Bean “A” which has a dependency of non-singleton (prototype) bean “B”.The container will create only one instance of bean “A” thus will have only one opportunity to inject the prototype bean “B” in it and every time you make a call to get bean B from bean A, always the same bean will be returned.
link: http://www.wideskills.com/spring/method-injection-in-spring#:~:text=Why%20do%20we,will%20be%20returned.
LOCS => overLOading - Compile time - Static binding
Comments
Post a Comment